General Instructions
In this homework: We are going to use Node and NPM to help us process markdown, file metadata, and render templates. This is in preparation to applying these techniques to out entire website in the next homework.
Create and Use a new Branch hw6
We will create a new git branch called hw6
(starting from your ‘hw5’ branch) for use in this assignment. The branch you create must exactly match the one I’ve given you for you to receive any credit for this homework.
Prior to creating this new branch make sure your working directory is “clean”, i.e., consistent with the last commit you did when you turned in homework 5. Follow the procedures in GitHub for Classroom Use to create the new branch, i.e., git checkout -b hw6
. Review the section on submission for using push with a new branch.
Note: Don’t branch from your midterm
branch. First git checkout hw5
then use the above command.
Use README.md
for Answers
You will modify the README.md
file to contain the answers to this homework.
# Homework #6 Solution
**Your name** **NetID: yourNetID**
Questions
Question 1. (15 pts) Node and NPM (installation), file read
Hints: all of the great JavaScript MDN: String, MDN: Array, Object, and more methods and functionality is available for use in Node.js. The HTML DOM and browser APIs are not! You will need to use a command console whose current working directory is your practice
directory to run the programs you write in this problem.
(a) Installation and Practice directory
Install node.js. Report the version number here. At the top level of your repo create a directory named practice
to hold your work for this homework. If you already have a similarly named directory in your repo remove any other files.
(b) Create an About.md Markdown File
Create an about.md
markdown file roughly based on your about.html
page for your club in your Practice
directory. Make sure it has some headings, and other content. Show a portion of that markdown file here (also commit the file).
(c) Simple File Read
Write a Node.js program to called processIt.js
to read the about.md
file and print out the number of lines in the file.
Show your program (JavaScript) here. Along with a screen shot of your command line running the program and showing its output.
My screenshot looks like.
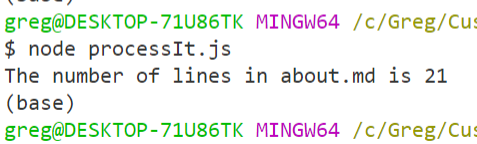
(d) Event Data in JSON
Which format is more restrictive: JSON or JavaScript? (Answer here)
Convert your JavaScript event data from the previous homework into a JSON file and put it in your practice
directory. You can check that you have proper JSON with an online JSON validator. Show your JSON here.
(e) JSON data import, console log
In your processIt.js
file program that use the line let events = require('./eventsFile.json');
to bring your JSON data file into a JavaScript array. Note that you do not have to separately read this file! Have this program print out the data from this file nicely without using any “for”, “do”, or “while” loops. Show your code here and show a screenshot of your console.
My screenshot looks like:
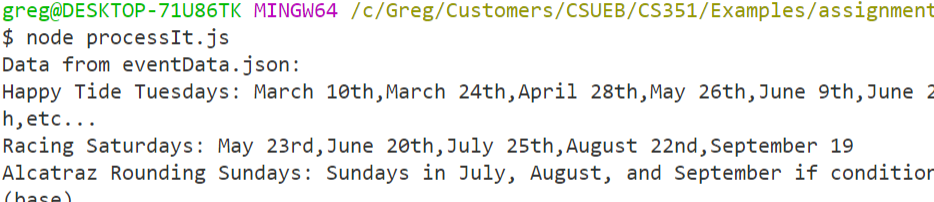
Question 2. (10 pts) Commonmark Package Install via NPM, File Read and File Write
Hints: You will need to use a command console whose current working directory is your Practice
directory. You will use this for installation and running your program.
(a) Create package.json
Within your Practice
directory create a package.json
file by one of the methods recommended in in the course slides. Be sure to commit this file to Git. Write the approach that you used to create the package.json
file here.
(b) Install Commonmark
We are going to use the commonmark open source package from NPM to write a JavaScript program to convert Markdown into HTML. Documentation can be found at NPM: Commonmark. We want to “save” this package dependency into our package.json
file so we will install the commonmark package by running the following command (from the command line while in the Practice
directory):
npm install --save commonmark
Show your package.json
file here (not the package-lock.json
).
(c) Read MD file and Process with Commonmark
Now add code to processIt.js
to process the about.md
file (string) with commonmark, and write it out to an HTML file. You should read the instructions at NPM: Commonmark but the essential code is:
let commonmark = require('commonmark');
let reader = new commonmark.Parser();
let writer = new commonmark.HtmlRenderer();
// Other stuff
let parsed = reader.parse(markdownDataString); // your markdown data in here
let result = writer.render(parsed); // result is an HTML string
Show your code here.
Show show a portion of the output HTML file from your program here.
Question 3. (10 pts) Meta data and YAML
We can use Commonmark to turn markdown into raw HTML but this doesn’t include page meta data, i.e., the stuff that goes into the header. In addition we may want to include meta data that will control how a template renders a page. A common method for attaching meta data to “partial HTML” or Markdown files is through a YAML header at the top of the file.
---
title: About the Windsurf Foiling Club
author: Dr. B.
description: The Bay area windsurf foiling club is my club!
---
(a) Install gray-matter
We are going to use a nice open source package called gray-matter to process this “front matter” at the beginning of the file. Install it for use in your Practice directory with the command:
npm install --save gray-matter
Add a YAML block with title, author, description information to the beginning of your about.md
file.
(b) YAML and Markdown Processing
To use gray-matter you need to “import” it with the
const matter = require('gray-matter');
To then process the data (after you read it) use:
let metaAndContent = matter(fileContents); // gives and object with content and data components
This produces a JavaScript object of the form {content: "string of file content", data: {}}
where the data
portion will have fields corresponding to the front matter items. Update your processIt.js
program to use gray-matter to separate the meta-data from the content. The content (markdown) should be processed with commonmark and for now console log the meta-data.
Show a screenshot showing the extracted metadata from the about.md
file. Mine looks like:
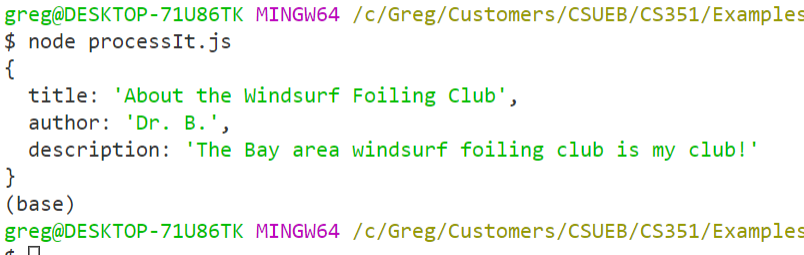
Show your updated processIt.js
file here.
Question 4. (15 pts) Nunjucks Templates
(a) Install and Setup Nunjucks
Within your practice
directory install Nunjucks (npm install --save nunjucks
). Create a views
directory within the practice
directory to hold your templates.
(b) Create Base Template
Similar to that shown in the Using Nunjucks create a base.njk template and menu for your site in the views
directory. Show your base.njk
template here.
You should have portions in the <head>
for title, author, and description that will be filled in from your YAML metadata. The <main>
content should come from processing your markdown files with Commonmark.
(c) Update processIt.js
to Render Template
Update your processIt.js
file to render your template based on the processed about.md
file. Save the output to a file called about.html
Show your updated processIt.js
here.
Show your generated about.html
here.