General Instructions
In this homework is to continue working with HTML, CSS, and JavaScript. In particular we will bring in images and emojis to our club site that we will deploy to an external server. We will continue our review of the JavaScript language and dynamically create and manipulate content via the DOM.
Create and Use a new Branch hw3
We will create a new git branch called hw3
for use in this assignment. The branch you create must exactly match the one I’ve given you for you to receive any credit for this homework.
Prior to creating this new branch make sure your working directory is “clean”, i.e., consistent with the last commit you did when you turned in homework 2. Follow the procedures in GitHub for Classroom Use to create the new branch, i.e., git checkout -b hw3
. Review the section on submission for using push with a new branch.
Use README.md
for Answers
You will modify the README.md
file to contain the answers to this homework.
# Homework #3 Solution
**Your name** **NetID: yourNetID**
Questions
Question 1. (15 pts) Images, Emojis, Selectors
1(a) Images
Add at least two images to your club website. One must be on your “home” page (index.html). Make sure your image is properly sized on the webpage, and that the image file itself is under 500KB. Put these images in an images
directory within the clubProject
directory (this is different from directory that you keep screenshots for you homework writeups!). Link to these images with relative URLs.
List the pages where you put the images here. Take a screen shot showing part of a page with an image on it and include it here. Here is an example from my club site:
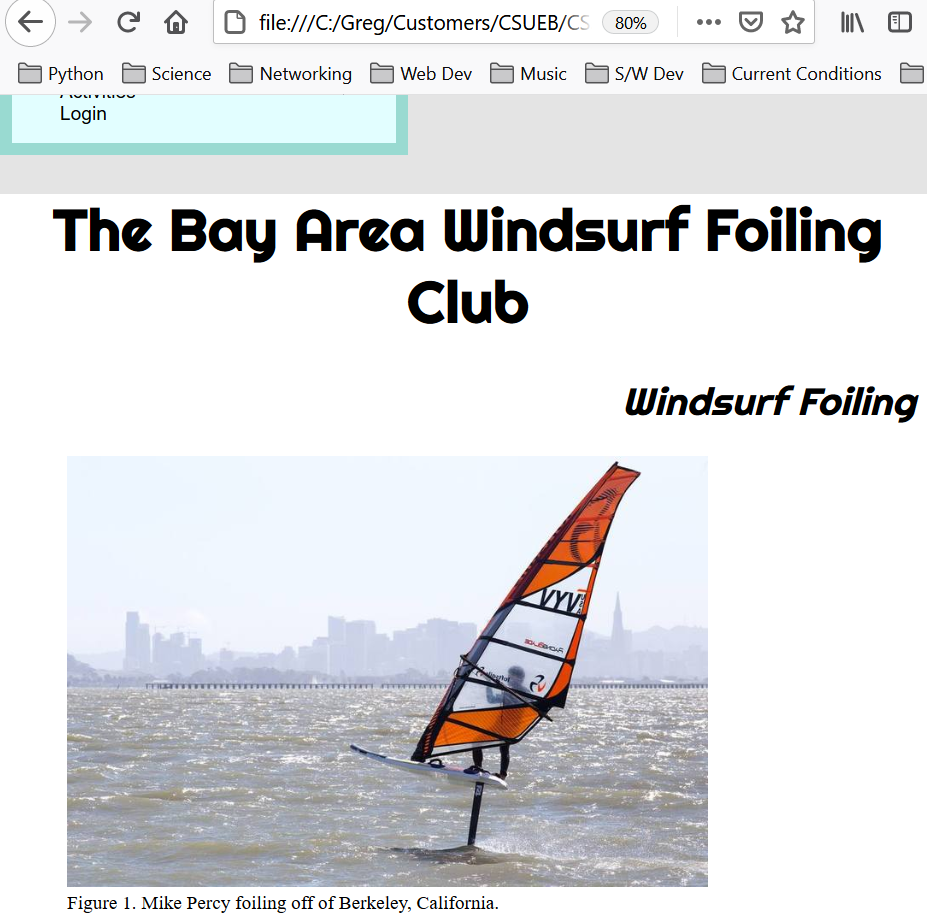
1(b) Emojis
Add a least two emojis to your club website via the character code method used in the class slides (and not via images, png, or SVG files).
Indicate the emojis you used here along with their character codes. Take a screen shot of part of a page showing at least one of the emojis that you used. My screen shot looks like:
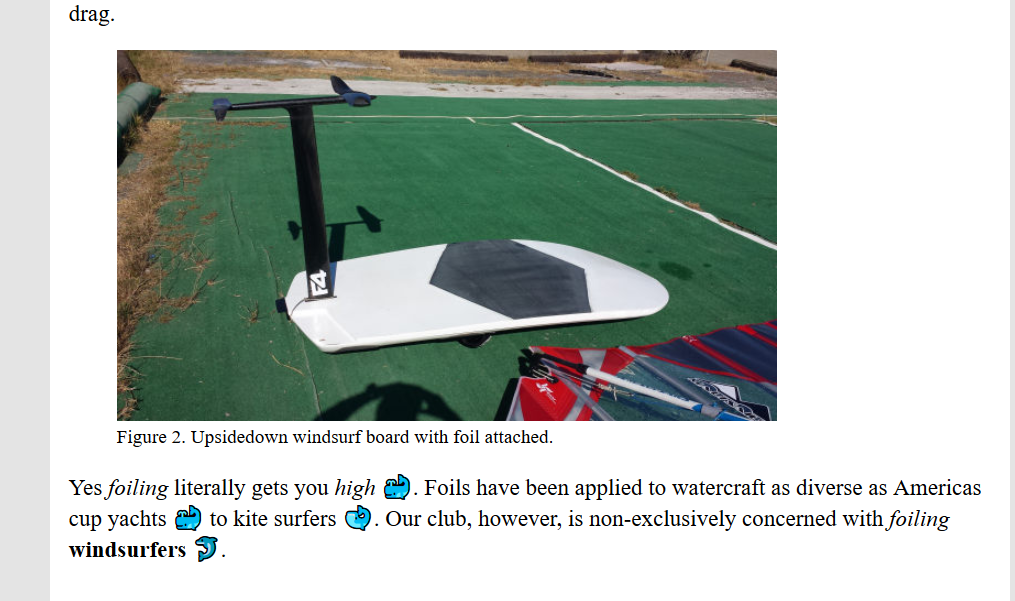
1(c) More Specific CSS Selectors
In the previous homework we applied styled our lists and links so that our page navigation would look like a “menu” rather than a list of links. However, this changed the styling for all lists <ul>
and all links <a>
on all pages and parts of our web site. Now that we have learned about descendant combinators we should restrict these style changes (from the default) to only those lists and links that are within a <nav>
. Update your CSS to only apply your list styling to the list in <nav>
and not any other list on the page. Show your updated CSS here.
Question 2. (15 pts) HTML Table for Activities & Table Styling
HTML tables are a very popular way to display data. See MDN Tables Tutorials, MDN Table Reference, and MDN Table Styling.
2(a) Empty Table with Heading
We will use a table to display club activities. At a minimum each of your club activities needs a date
and description
properties which will translate to table columns. You can have more properties for an activity and hence more table columns.
A table element <table>
can contain <caption>
, <thead>
(table heading), and <tbody>
elements and more per the reference above. Add an empty table unstyled table with column headings to your page.
2(b) Table Rows with Sample Info
Add at least three rows to your table with example activities. At this point my unstyled table looks like:
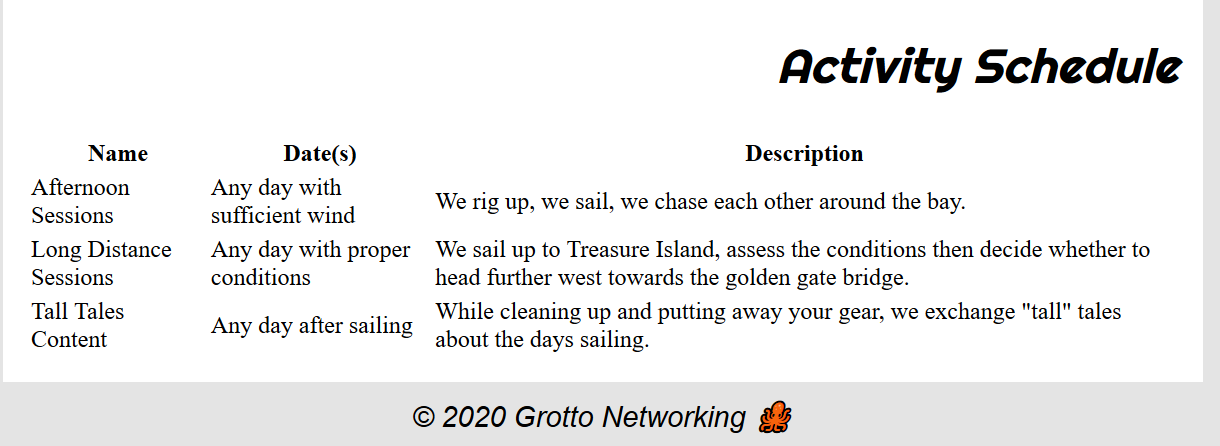
Show the HTML for your table here (nicely syntax highlight via Markdown)
2(c) Style Table
Style your table in a reasonable way (put the styling in your club.css
). Take a screenshot and show just the CSS for your table here.
My screenshot looks like:
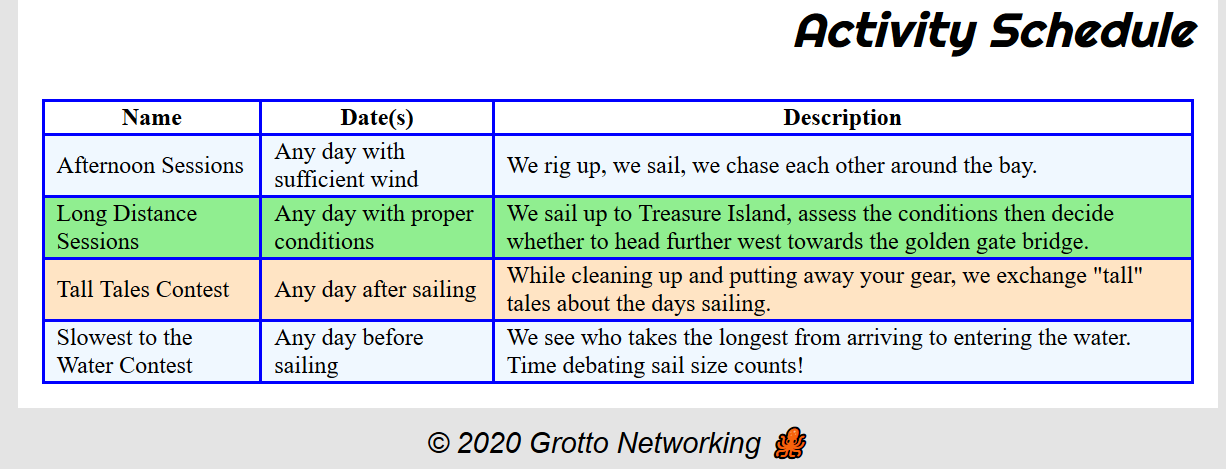
Note: I added another row to demonstrate my “triple tiger striping” styling. I tried a demo of this on the fly in the topic video on selectors but made a mistake and removed that section from the video.
Question 3. (10 pts) Server Deployment
Deployment of web pages. I’ve put the basic login instructions from the department on Blackboard. Please try it early and come to me with any questions. You may use any FTP client you want to transfer files from your computer to your account on the server. I’ve used FileZilla which available for Windows, Mac, Linux. The settings I used in Filezilla are shown below and should apply to other FTP clients (use your own account information!):
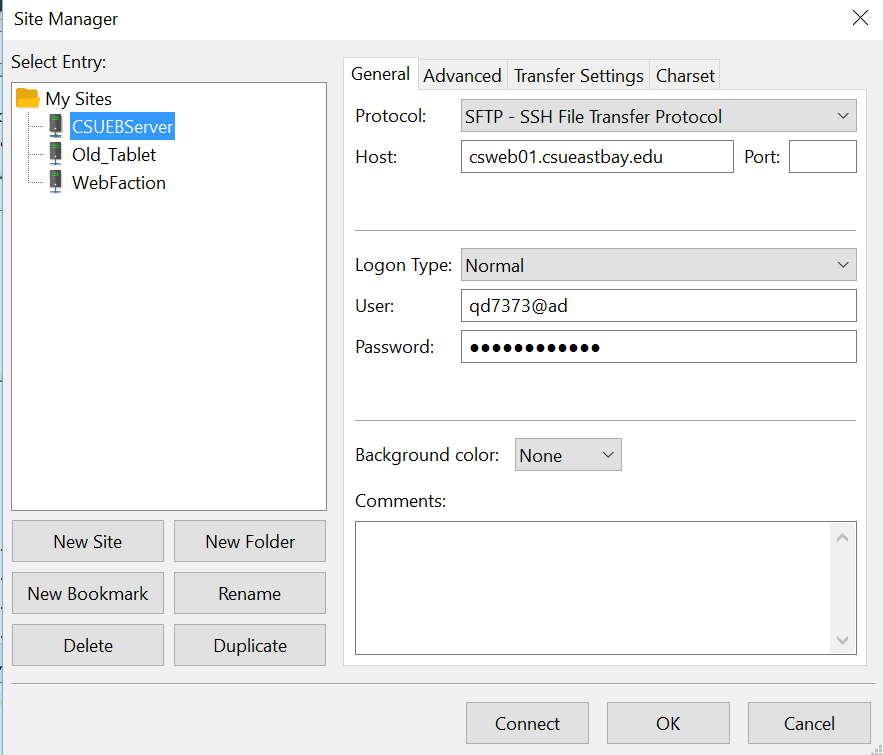
3(a) File Transfer
Using Filezilla or any other FTP transfer program transfer your entire clubProject
directory to the public_html
directory. You may want to change the directory name on the server to clubProjectHW3
to keep your different deployments separate. You should then be able to visit your website at: http://csweb01.csueastbay.edu/~YourNetId/yourProjDirName/.
Where you should substitute your NetId and club project directory name. Take a screenshot showing you club’s home page and the URL in the browser window. Mine looks like:
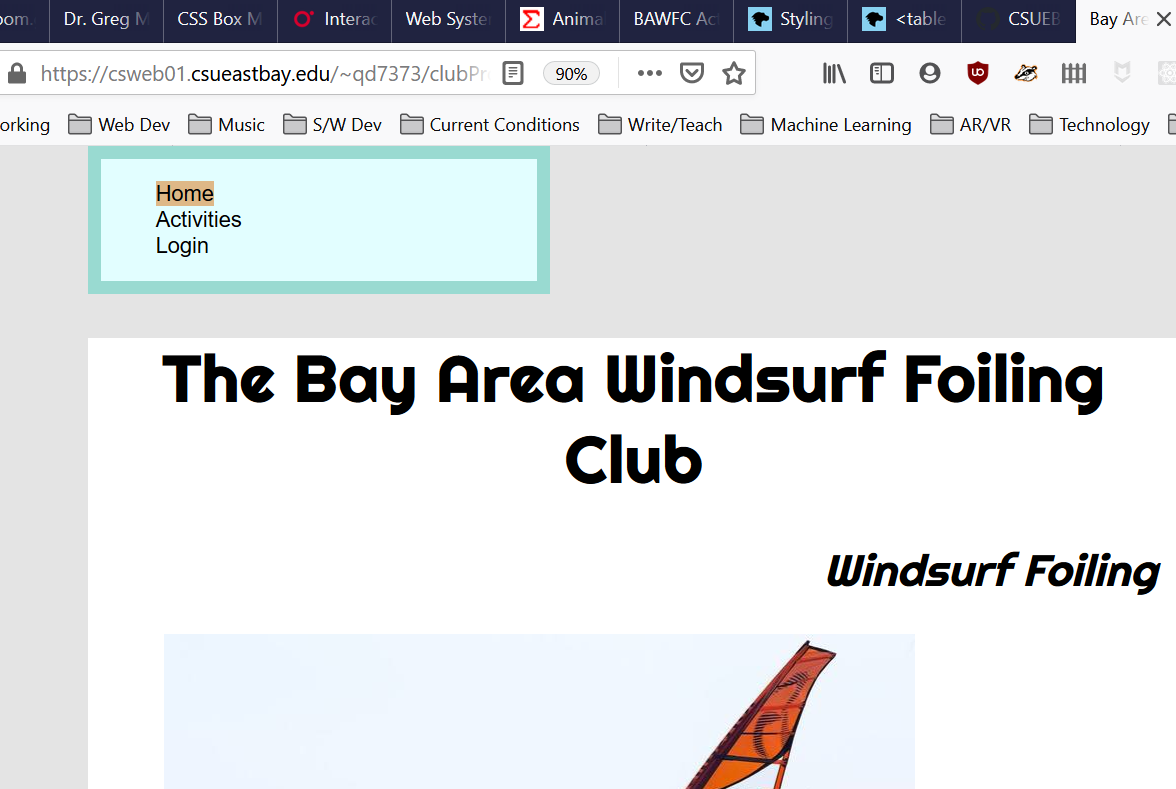
3(b) Testing
Test all the links (pages) and make sure your site works, in particular check for broken links, missing images, and styling problems. Fix any issues to receive full credit.
Put the URL to your club site here. Warning: This link must work to receive any credit for this problem.
Question 4. (10 pts)
JavaScript: Arrays and basic Objects. MDN Array
(a) Array basics
JavaScript arrays are really lists in that they can grow and shrink and can contain any kind of element including a mix of different types of elements. Let’s try some basic array manipulations. Provide answers to the questions in the comments and a partial screen shot of your debug console.
= "A long, long, time ago in a galaxy"; // A string
sentence = sentence.split(' '); // (i) what does this do?
myArray console.log("The number of words is: " + myArray.length);
.push("far"); // (ii) what do each of these calls do?
myArray.push("far");
myArray.push("away");
myArrayconsole.log("The number of words is: " + myArray.length);
console.log(myArray.join('_')); // (iii) what does join do?
= myArray.indexOf('galaxy'); // (iv) What does this do?
tempIndex = "college"; // (v) did I change an element?
myArray[tempIndex] console.log(myArray.join(' '));
.pop(); // (vi) what does this do?
myArrayconsole.log("The number of words is: " + myArray.length);
(b) Fancier Array Methods
In many cases in JavaScript we provide a function as a parameter to another function. This is a kind of “functional programming” and we need to get used to it. We are going to try this with the map and sort array methods. Hint: you will find the answers to most of the questions below by looking at the MDN Array documentation.
= "Whereas recognition of the inherent dignity and of the equal and inalienable rights of all members of the human family is the foundation of freedom, justice and peace in the world";
sentence2 = sentence2.split(" ");
myArray2
function upper(xString) { // (i) What does this function do?
return xString.toUpperCase();
}
= myArray2.map(upper); //(ii) What does map do?
myArray3
function noCaseSort(x, y) {
if (x.toLowerCase() < y.toLowerCase()) { // (iii) why would I do this?
return -1;
else {
} return 1;
}return 0;
}
.sort(noCaseSort);
myArray2// (iv) what is the purpose of passing this function here?
(c) Objects
JavaScript Objects are extremely versatile and relatively easy to use. So much so that we will not need to define any object instances via JavaScript classes until the end of the course when we work with React. Let’s see how this works. Substitute your information in the following and run it in the developer console. Click to expand some of the objects fields and take a screen shot for your answer.
= {}; // creates an empty object
me .name = "Your name here";
me"favorite desert"] = "Your favorite desert here";
me[// (i) Why this syntax and not that used for the name field/property
.courses = ["CS351", "Your other courses"];
me// (ii) can I put an array in an object?
.major = "Your major";
me.number = 42; // (iii) your favorite number
me.codeEditors = ["Notepad++", "Brackets", "Atom"]; // (iv) Put in yours here
me"nick name"] = "Dr. B";
me[console.log(me);