General Instructions
The goals of this assignment are to get familiar with AES, Public key Cryptography, Hash functions, and Digital Signatures.
Create and Use a new Branch hw8
We will create a new git branch called hw8
for use in this assignment. The branch you create must exactly match the one I’ve given you for you to receive any credit for this homework.
Prior to creating this new branch make sure your working directory is “clean”, i.e., consistent with the last commit you did when you turned in homework 7. Follow the procedures in GitHub for Classroom Use to create the new branch, i.e., git checkout -b hw8
. Review the section on submission for using push with a new branch.
Use README.md
for Answers
You will modify the README.md
file in your repo to contain the answers to this homework.
Questions
Question 1. (10 pts) AES
Refer to Wikipedia: Block Cipher Modes of Operation and the course slides to answer the following.
(a) When and Why?
When and why must you use a “block cipher mode of operation” with AES (besides electronic code book ECB)?
(b) AEAD
What do “AEAD” modes provide in addition to confidentiality? Use terminology from the CIA triangle.
(c) Mode Choice
Of the “confidentiality” modes, why would might you consider counter (CTR) mode over cipher block chaining (CBC) mode.
(d) Disk Encryption
See Wikipedia: Disk Encryption Theory. Does disk encryption pose special constraints? If so list two? Is the potential adversary situation for disk encryption more complicated than that of communications with a website? If so give examples of “strong adversary capabilities” considered for disk encryption not considered in web communications.
Question 2. (10 pts) Public Key
(a) Public Key Short Answer
Answer the following:
- Which algorithms are faster for bulk encryption symmetric (like AES) or public key (like RSA)?
- Given N people that want to communicate securely how many keys need to be shared so that everyone can communicate with symmetric encryption?
- Given N people that want to communicate securely how many keys need to be shared so that everyone can communicate with public-key encryption?
- Sharing keys securely is easy to do? (True/False)
(b) Key Exchanges
Like in the course notes you will perform a key exchange using public key cryptography to yield a shared secret key for use in symmetric cryptography. See X25519 key exchange.
Use the following Python imports:
from cryptography.hazmat.primitives.asymmetric.x25519 import X25519PrivateKey
from cryptography.hazmat.primitives import serialization
Also use code like the following to print out public keys in a standard text format:
print(bob_public_key.public_bytes(encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo))
Do and answer the following:
- Generate X25519 private and public keys for a user named Alice. Show Alice’s public key here in standard format.
- Generate X25519 private and public keys for a user named Bob. Show Bob’s public key here in standard format.
- Have Alice generate a secret key for use with Bob. Which of Bob’s key’s should key get and use. Use the X25519 key exchange algorithm. Show the hex for this secret key.
- Have Bob generate a secret key for use with Alice. Which of Alice’s key’s should key get and use. Use the X25519 key exchange algorithm. Show the hex for this secret key.
- Are the symmetric secret keys that Alice and Bob generated the same?
- How long are the symmetric secret keys generated? Which symmetric algorithm can use a key of this length?
(c) WireGuard
What is WireGuard? What algorithm does WireGuard use to generate a symmetric key from public/private pairs? How does it get these keys?
Question 3. (10 pts) Secure Hashes and HOTP
(a) Secure Hashes
As in the course notes compute the SHA256 hash of a text file. It can be any file but it needs to be longer than 3kB. Change one character in the file. Recompute the hash. Change that character back to its original value and recompute the hash.
Show the following:
- Name of the file and its length
- Original hash of the file in hex.
- Hash of the modified file in hex.
- Hash of the file after you undid the change.
(b) Base32
What is Base32 encoding and why would one use it?
(c) HOTP
Using either the PyOTP library or any RFC4226 compliant library and language of your choice. Use the following Base32 encoded secret key “CSSIXSEVENTYONEUSINGONEKEYFORALL”. Generate the nth iteration of the HOTP algorith where n is the last four digits of your NetId.
Question 4. (10 pts) Signatures and Certificates
(a) Digital Signatures
As in the course slides you will use Elliptic curve public key cryptography to (securely) sign and verify a message. Reference ED25519.
Do and answer the following:
- Create Ed25519 private and public keys.
- Show your public key in a standard text format here.
- Create a simple text message (it must be different from mine in the class notes)
- Generate a signature with Ed25519. How long is the signature? Which key was used to generate the signature.
- Verify the signature using the Ed25519 algorithm. Which key did you use to verify the signature?
- Modify the message by one character. Try to verify the modified message against the signature.
Show your Python code here.
(b) Certificates
Visit a website supporting HTTPS (Chrome is easiest to get certificate information from) and answer the following questions about the X.509 certificate for the website:
- Who is the certificate issued to?
- What is the certification path, i.e., who signed the certificate
- What is type and length of the public key
- What signature algorithm was used?
- What are the validity dates?
Question 5. (10 pts) TLS
(a) WireShark and TLS Capture
Use WireShark to capture the TLS handshake when you open a new browser tab or window to a website different from what I showed in class. Then do and answer the following:
- Take a screenshot of the Client Hello packet. I get something like:
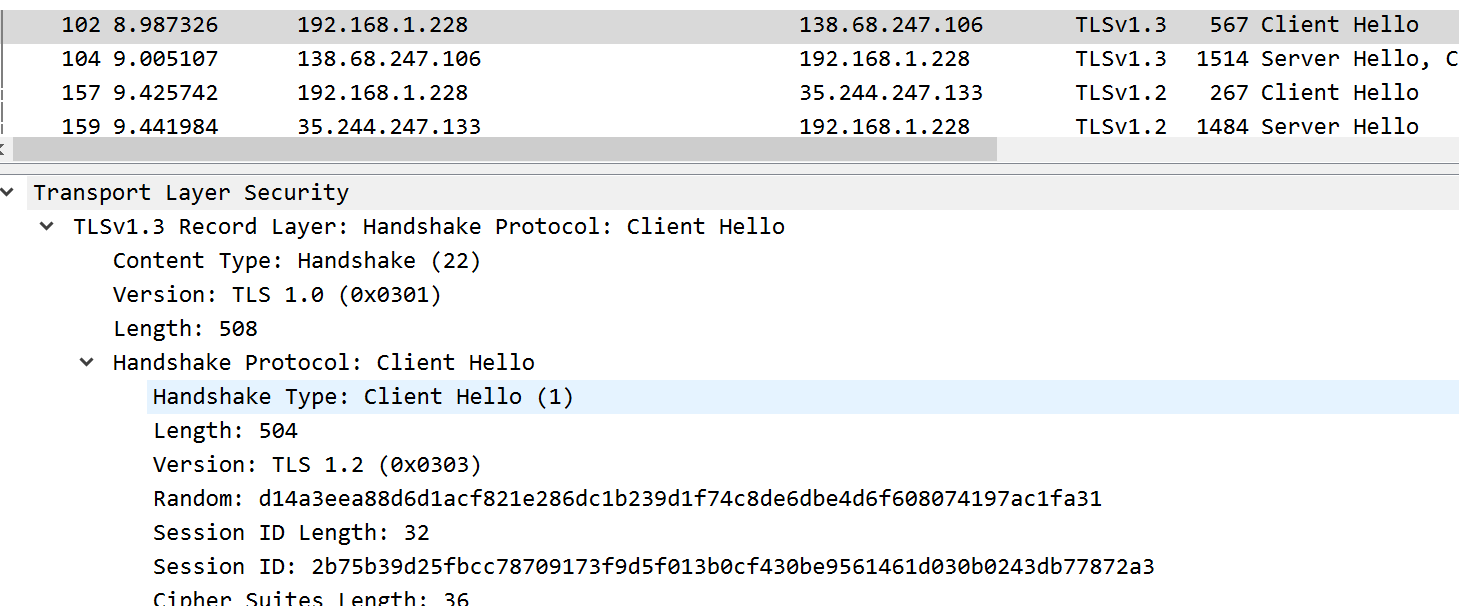
(b) Cipher Suites
How many different cipher suites is you client willing to accept? List the first five here.
(c) Server Hello
Take a screenshot of the Server Hello packet. I get something like:
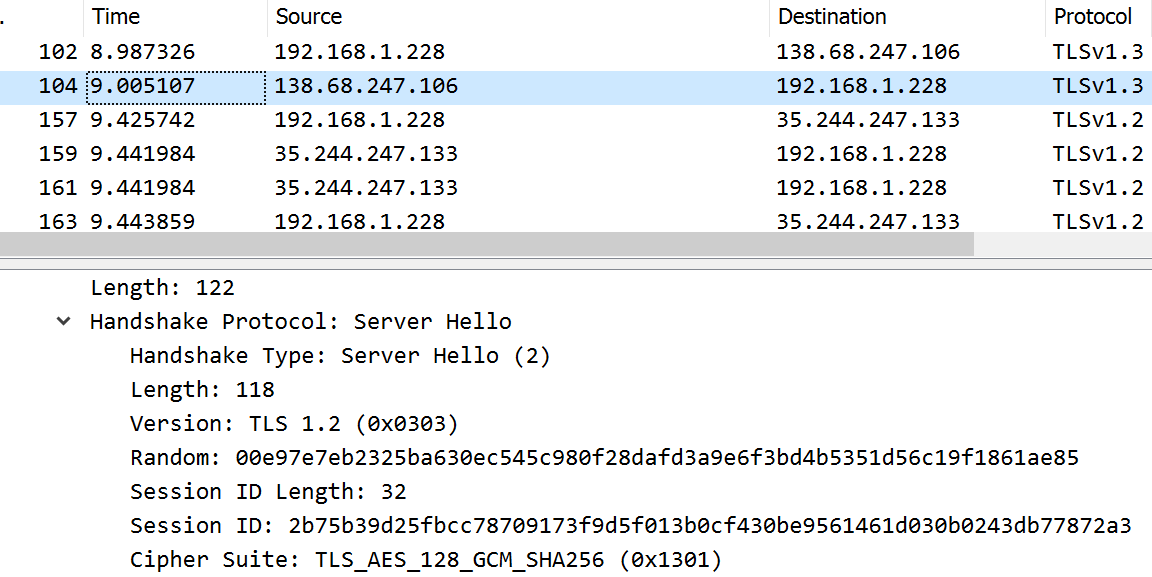
(d) Cipher Agreement
What cipher suite did the client and server decide to use? In particular what was the block cipher? What was the block cipher mode? And what was the cryptographic hash algorithm?